Kotlin is a powerful programming language that is particularly beneficial for beginners, making it an ideal choice for those who are new to coding. Furthermore, this language simplifies the understanding of variables by pairing them with straightforward data types, which are essential in programming. Consequently, by mastering these basics, you can begin coding effectively in Kotlin.
What Are Variables in Kotlin?
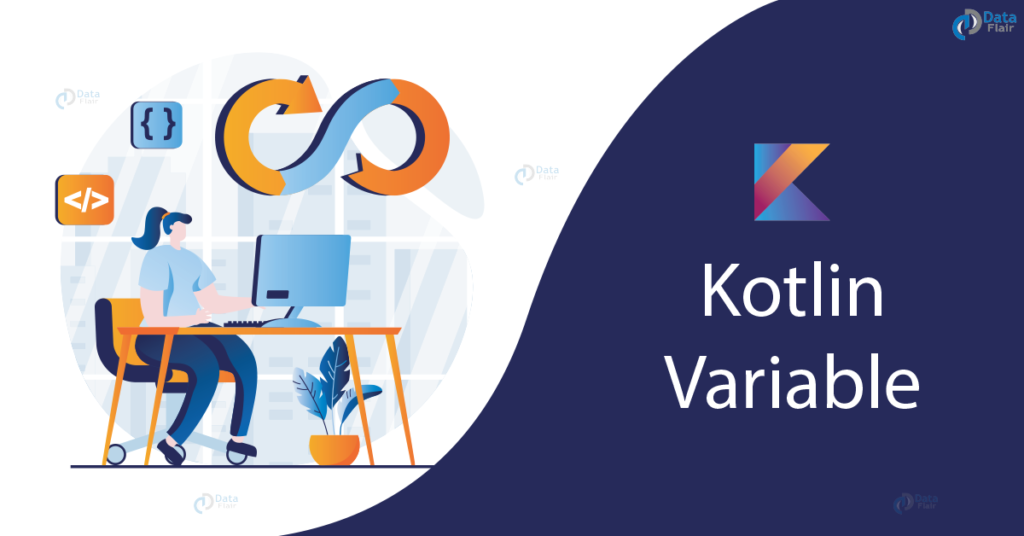
In the world of programming, variables serve as fundamental constructs that allow you to store data for later use. Specifically, when working with Kotlin, you have the ability to declare variables using either val for read-only variables or var for mutable ones. For instance, an Int type variable can store an integer value.
Declaring Variables in Kotlin
To declare variables in Kotlin, you can choose between val or var, depending on whether you need the variable to be immutable or mutable. Below is an example to illustrate:
val pi: Double = 3.14159 // Immutable variable, cannot be changed
var counter: Int = 10 // Mutable variable, can be changed
// Trying to change `pi` will result in a compile-time error
// pi = 3.14 // Uncommenting this line will cause an error
counter = 15 // This is allowed, `counter` is mutable
Explanation:
• The pi variable, declared as Double, is immutable because it uses val.
• On the other hand, the counter variable, declared as Int, is mutable and can be modified as it uses var.
Understanding Kotlin Data Types
Kotlin supports a variety of data types, each serving a specific purpose. By leveraging these types, you can ensure that your variables store the correct kind of data. Moreover, these data types are crucial for performing operations effectively in Kotlin.
Basic Data Types in Kotlin
Let’s explore some of the fundamental data types available in Kotlin:
val age: Int = 25 // Integer type
val temperature: Double = 36.6 // Double type (floating-point)
val isKotlinFun: Boolean = true // Boolean type
val greeting: String = "Hello, Kotlin!" // String type
These data types are fundamental to performing various operations in Kotlin, ensuring that the right kind of data is stored in each variable.
Examples and Best Practices for Variables and Data Types
Let’s delve into some practical examples and best practices when working with Kotlin variables and data types.
Example 1: Working with Numeric Types
Kotlin enables arithmetic operations with numeric types while maintaining type safety, making it easier to manage numeric data.
val num1: Int = 10
val num2: Int = 20
val sum: Int = num1 + num2 // Simple addition
val average: Double = (num1 + num2) / 2.0 // Calculating average, note the division by 2.0
Explanation:
• The sum variable stores the result of adding num1 and num2, both integers.
• Meanwhile, the average variable is a Double because dividing by 2.0 ensures the result is a floating-point number.
Example 2: Using Strings in Kotlin
Strings in Kotlin are versatile and allow for various manipulations, providing flexibility in handling text.
val firstName: String = "John"
val lastName: String = "Doe"
val fullName: String = "$firstName $lastName" // String interpolation
val message: String = "Hello, $fullName! Welcome to Kotlin."
println(message) // Output: Hello, John Doe! Welcome to Kotlin.
Explanation:
• Kotlin supports string interpolation, which allows you to embed variables directly within strings using the $ symbol.
• The fullName combines firstName and lastName into a single string.
Example 3: Handling Boolean Logic
Boolean operations are fundamental in controlling program flow.
val isAdult: Boolean = age >= 18 // Checks if age is 18 or more
val isEligible: Boolean = isAdult && isKotlinFun // Both conditions must be true
if (isEligible) {
println("You are eligible and find Kotlin fun!")
} else {
println("Eligibility criteria not met or you don't find Kotlin fun.")
}
Explanation:
• isAdult is a Boolean that checks if the age is 18 or older.
• isEligible uses the logical AND operator (&&) to combine two Boolean expressions.
Best Practices for Working with Variables and Data Types in Kotlin
Following best practices when writing Kotlin code ensures that your code is robust, readable, and maintainable:
• Use Descriptive Names: Variable names should clearly reflect their purpose. Avoid single-letter names, except for trivial cases like loop indices.
• Prefer val Over var: Whenever possible, use val to declare variables. This practice makes your code more predictable and reduces the likelihood of bugs due to unintended variable reassignment.
• Type Inference: Kotlin can infer the type of a variable. If the type is obvious, you can omit it for brevity. However, in more complex cases, explicitly specifying the type can improve readability.
val height = 180 // Type inferred as Int
val weight = 75.5 // Type inferred as Double
Conclusion – Wrapping It All Up
Understanding variables and data types is fundamental to mastering Kotlin, or any programming language. This guide provided a comprehensive look at how to declare and use variables, work with various data types, and follow best practices. With these basics under your belt, you’re now equipped to start exploring more advanced Kotlin concepts and build your own projects.
For more insights into Kotlin, check out our Kotlin Programming category and other related articles on Kotlin Coroutines.