Developers have widely used Java as a powerful programming language, making it essential in software development since Sun Microsystems launched it in 1995. Therefore, understanding Java OOP Basics is crucial for any developer who wants to write effective Java code. Known for its “write once, run anywhere” capability, You can run Java programs on any device with a Java Virtual Machine (JVM), which makes it a versatile choice for various platforms.
Oracle Corporation acquired Sun Microsystems in 2010 and took over Java’s development and stewardship. Oracle has guided Java’s evolution, providing regular updates with significant enhancements in performance, security, and new features like lambdas and the Stream API introduced in Java SE 8.
With this understanding of Java’s history and its current stewardship under Oracle, let’s explore the core concepts of Object-Oriented Programming (OOP) in Java. If you’re interested in more Java-related topics, don’t forget to check out our Java Programming category.
What is Object-Oriented Programming?
Object-Oriented Programming (OOP) is a programming paradigm that allows developers to structure programs in a more logical and organized manner. But what exactly is OOP? OOP is a way of organizing a program around “objects,” which represent real-world entities. These objects have properties (attributes) and behaviors (methods). Understanding Java OOP Basics is crucial for any developer who wants to write effective Java code. In this guide, we’ll cover the key Java OOP Basics, including classes, objects, inheritance, polymorphism, and encapsulation.
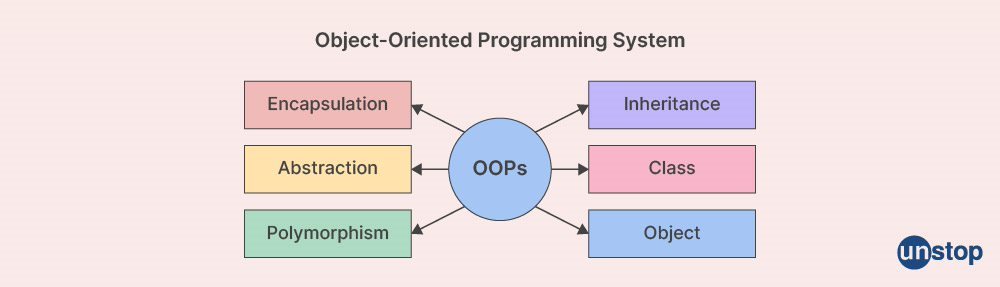
Understanding Classes and Objects in Java OOP Basics
In fact, in Java, everything begins with a class and an object. Classes are blueprints that define how objects are created and how they behave. Objects are instances of classes, representing specific elements in your program.
Imagine you’re designing a car. In Java, you can represent a car by creating a Car class. This class can define properties like the car’s model and color, as well as behaviors like starting the engine.
// A simple example of a Car class
public class Car {
// Properties
String model;
String color;
// Behaviors
void startEngine() {
System.out.println("Engine started.");
}
}
Explanation: In this example, the Car class stores the car’s model and color. The startEngine method is used to start the car’s engine.
You can then create various car objects (instances of the Car class) like this:
// Creating an object from the Car class
Car myCar = new Car();
myCar.model = "Toyota";
myCar.color = "Red";
myCar.startEngine(); // Output: Engine started.
Here, myCar is a Car object, and you can assign values like model and color to it.
The Four Pillars of Java OOP Basics
OOP in Java is built on four main principles: Classes and Objects, Inheritance, Polymorphism, and Encapsulation. Understanding these four principles is key to writing effective Java code.
Inheritance in Java OOP Basics
Inheritance allows one class to inherit properties and behaviors from another class. This enhances code reusability and simplifies the maintenance of your program. For example, if you want to create an ElectricCar class, you can inherit from the Car class:
// The ElectricCar class inherits from the Car class
public class ElectricCar extends Car {
int batteryLife;
void chargeBattery() {
System.out.println("Battery charging...");
}
}
Explanation: The ElectricCar class inherits all properties and behaviors from the Car class and adds new features like batteryLife and the chargeBattery method.
Polymorphism – One Interface, Many Implementations
Moreover, polymorphism is one of Java’s most powerful features. It allows objects to take on different forms. For example, the same method name can have different implementations in different classes.
public class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
public class Dog extends Animal {
@Override
void sound() {
System.out.println("Dog barks");
}
}
public class Cat extends Animal {
@Override
void sound() {
System.out.println("Cat meows");
}
}
Explanation: Here, the Dog and Cat classes both inherit from the Animal class. Each class overrides the sound method to provide a specific implementation.
Encapsulation – Protecting Your Data
Encapsulation allows you to hide a class’s internal details from the outside world. In other words, the class’s data members (attributes) are usually declared as private, and access is provided through methods within the class. This protects the data and prevents misuse.
public class BankAccount {
private double balance;
public void deposit(double amount) {
if(amount > 0) {
balance += amount;
}
}
public double getBalance() {
return balance;
}
}
Explanation: The BankAccount class keeps the balance variable private. This means it cannot be accessed directly; instead, it is controlled through the deposit and getBalance methods.
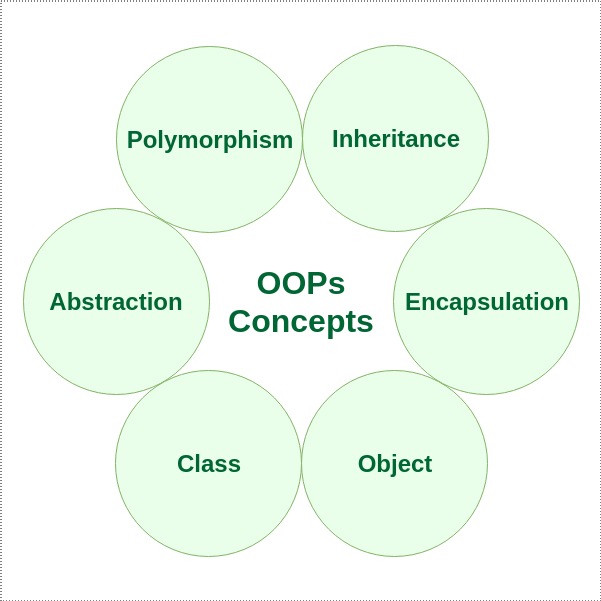
Conclusion – Wrapping It All Up
Object-Oriented Programming forms the foundation for writing effective Java code. By understanding the basic OOP concepts of classes and objects, inheritance, polymorphism, and encapsulation, you can create software that is more manageable, secure, and flexible. With the knowledge from this article, you are now ready to start building your own Java projects using OOP principles.