Introduction
Swift, introduced by Apple in 2014, is a modern programming language designed for developing applications on platforms like iOS, macOS, watchOS, and tvOS. It offers significant advantages for developers looking to create robust and efficient apps within the Apple ecosystem. In this article, we will explore the fundamental concepts of Swift and provide a solid foundation for those who want to start building projects with Swift.
Key Features of Swift
Swift stands out among other programming languages due to its focus on security, speed, and flexibility. Developed by Apple, it plays a crucial role in modern software development. Here are some of the key features that make Swift unique:
- Security: Swift automatically checks for errors in data types, minimizing programming mistakes and allowing for more secure code.
- Performance: Swift is significantly faster than Objective-C, thanks to Apple’s LLVM compiler technology, which enhances application performance.
- Modularity: Swift allows you to write code in modular structures, making your projects more manageable and sustainable, especially in larger applications.
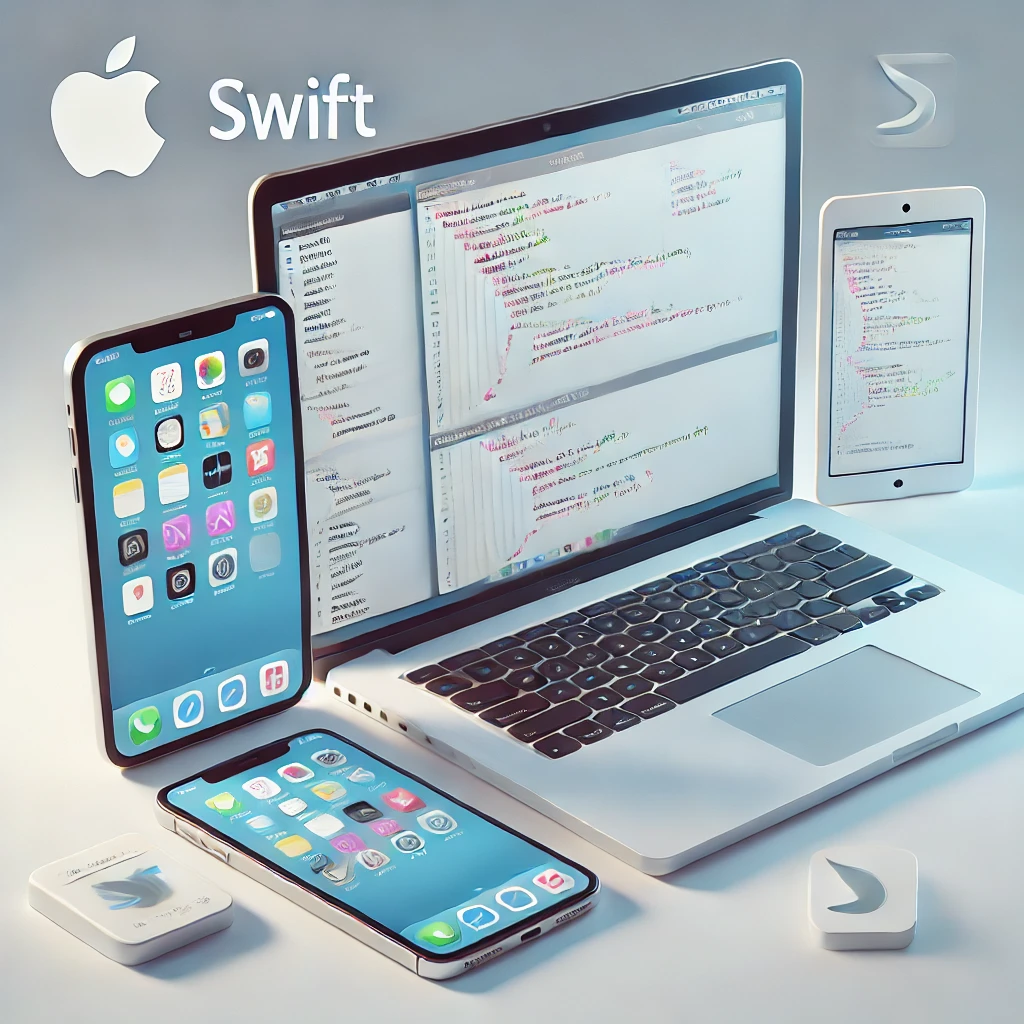
For more information, visit the Swift Programming Language official page by Apple.
Writing Your First Swift Code
Getting started with Swift is straightforward. Below is a “Hello, World!” example that demonstrates how user-friendly the language is:
import UIKit
var greeting = "Hello, World!"
print(greeting)
This simple example shows how to define a basic variable in Swift and print it to the console, making it an ideal starting point for beginners.
Variables and Constants
In Swift, data is stored using variables and constants. Variables are defined with the var
keyword, while constants are defined with the let
keyword:
var name = "Ahmet"
let age = 25
In this example, name
is defined as a variable, while age
is a constant. Constants cannot be changed after their initial assignment, which adds security to your code. For more detailed information, explore articles in the Swift category on birdeveloper.com.
Data Types in Swift
Swift strictly controls data types, ensuring that every variable has a specific type. This adds a layer of security to your code. Commonly used data types in Swift include:
- Int: Whole numbers.
- Double and Float: Decimal numbers.
- String: Text strings.
- Bool: Boolean values (true or false).
Example:
let number: Int = 10
let pi: Double = 3.14
let isSwiftAwesome: Bool = true
Control Flow in Swift
Swift provides essential control flow structures like if-else statements and loops to manage the flow of your program.
if-else Structure:
let score = 85
if score >= 90 {
print("Congratulations, you got an A!")
} else if score >= 80 {
print("Well done, you got a B!")
} else {
print("Keep working hard!")
}
Loops:
Swift supports both for
and while
loops. Here’s an example of a for
loop:
This loop prints numbers from 1 to 5 to the console, illustrating how easily loops can be implemented in Swift.
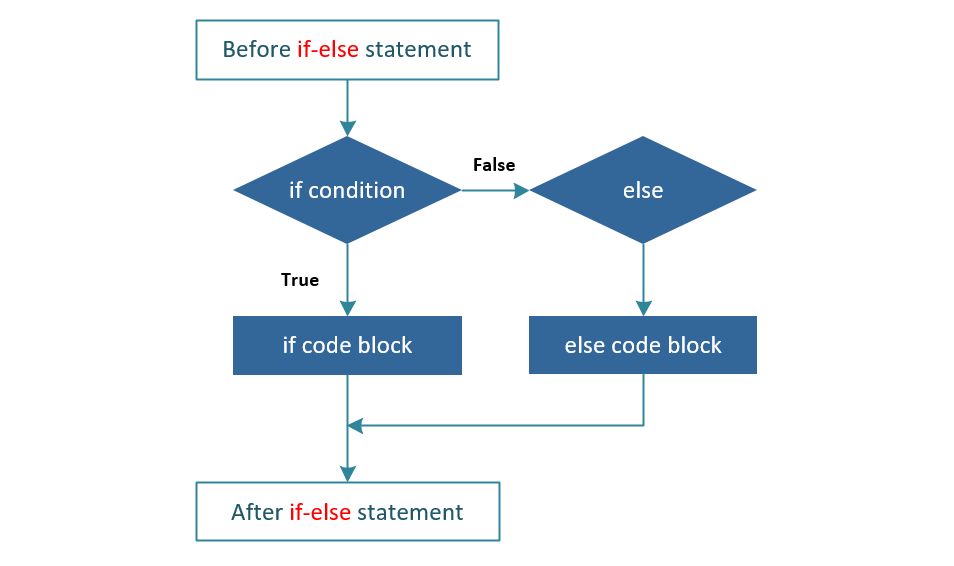
Functions in Swift
Functions are blocks of code in Swift that perform specific tasks. They help make your code more modular and reusable.
func greet(name: String) -> String {
return "Hello, \(name)!"
}
let greetingMessage = greet(name: "Ahmet")
print(greetingMessage)
This function takes a name as input and returns a greeting message. Using functions in Swift helps keep your code organized and easy to understand.
Taking Your First Steps with Swift
Swift, with its modern structure and integration within the Apple ecosystem, offers an excellent starting point for software development. With these foundational concepts, you can begin developing projects in Swift right away. Remember, continuous learning is essential in the software world, and Swift will be a valuable tool on this journey.